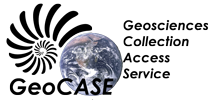 |
Simple ABCDEFG provider access portal |
Important Note:
This is a simple data portal for the GeoCASe network. It uses BioCASe technology to perform a distributed query on ABCDEFG providers, using the BioCASE protocol. Since searches are performed on the orginal provider's databases, they might take some time and yield less results than expected, if some of the providers are offline.
|
<%@page import = "java.util.logging.*" %>
<%@page import = "java.util.*" %>
<%@page import = "java.text.SimpleDateFormat" %>
<%@page import = "unitloader.*" %>
<%@page import = "unitrequestor.*" %>
<%@page import = "queryextender.*" %>
<%@page import = "translator.*" %>
<%@page import = "taxoRef.*" %>
<%@page import = "java.io.*" %>
<%@page import = "java.sql.*" %>
<%@page import = "javax.xml.transform.*" %>
<%@page import =" javax.xml.transform.stream.*" %>
<%
/* ---------------------------------------------------------------------------------------- */
/* Version 1.1 */
/* Authors: a.guentsch, a.hahn, j. de la torre, j. holetschek, m.doering */
/* REVISION: $Id: UnitLoader.jsp,v 1.5 2005/06/23 12:18:56 markus Exp $ */
/* ---------------------------------------------------------------------------------------- */
/* requires: */
/* - a JDBC Driver for SQL-Server (mssqlserver.jar in /WEB-INF/lib) */
/* - a JDBC Driver for PostgreSQL (pg73jdbc2.jar in /WEB-INF/lib) */
/* (on linux moved to $JAVA_HOME/lib/ext */
/* - the libraries jaxen-full.jar, jdom.jar, msbase.jar, msutil.jar, saxpath.jar */
/* in /WEB-INF/lib */
/* - the configuration file "consultantDBs.xml" in /WEB-INF */
/* - the queryextender, unitloader and unitrepquestor-classes to be stored in */
/* /WEB-INF/classes */
/* - the queryconcepts.xml, operators.xml and configuration.xml- files in */
/* /WEB-INF/classes/unitloader */
/* ---------------------------------------------------------------------------------------- */
/* known issues and problems: */
/* - the country field is free text entry - it should be replaced with */
/* a combobox offering country names and operating on ISO codes in the */
/* "background" (22.8.03, a.hahn) */
/* ---------------------------------------------------------------------------------------- */
/* -------------------------- CONSTANTS --------------------------------------------------- */
/* These constants give identifier strings for consulted external sources. */
/* Their connection details have to be defined in 'consultantDBs.xml' */
/* ---------------------------------------------------------------------------------------- */
final Level LOG_LEVEL = Level.ALL;
String CONFIGFILENAME = application.getRealPath("/") +"WEB-INF/conf/consultantDBs.xml";
final String TRANSFORMER_NAME = "en_ABCD2.0_All_parts.xslt";
final String TAX_EXTENDER_DB = "EM_bgbm22";
final String LOGGING_DB = "corm_write";
/* --------------------- USER INPUT AND PASSED PARAMETERS --------------------------------- */
/* get FullName, ISOCode and email parameters posted from form */
/* - concepts have to be registered in: /unitloader/queryconcepts.xml */
/* the request for a scientific name should always be automatically extended - double ** */
/* must be avoided(turns up in the Request Protocol string and leads to inacurate queries) */
/* ---------------------------------------------------------------------------------------- */
// allow user to limit the maximum number of results per provider and waiting time
int timeout = 30000;
int waitBeforeFetch = 15000;
int maxResultNo = 10;
try {
maxResultNo = Integer.parseInt(request.getParameter("returnLimit"));
waitBeforeFetch = Integer.parseInt(request.getParameter("TimeLimit")) * 1000;
timeout = waitBeforeFetch;
}
catch (Exception e) {
}
//Intialize Taxonomic Referencing System
taxoRef taxonomicReference = new taxoRef();
//Initialize ArrayLists Taxonomic Referencing System
ArrayList paleoNameSynonyms = new ArrayList();
ArrayList higherTaxonSynonyms = new ArrayList();
//Initialize Translation ArrayLists
ArrayList translationsCountry = new ArrayList();
ArrayList translationsHigherTaxon = new ArrayList();
/* Initialize TranslationsParsers */
TranslationsParser translationsParserIsoCountry = new TranslationsParser();
TranslationsParser translationsParserHigherTaxon = new TranslationsParser();
/* Get synonyms from Paleobiology Database (http://pbdb.org) */
if ((!paleoName.equals("*")) && (TRSynonyms != null)) {
String searchTerm = paleoName;
searchTerm = searchTerm.replace("*", "");
searchTerm = searchTerm.replaceAll(" ", "%20");
paleoNameSynonyms = taxonomicReference.getTaxonomicSynonyms("http://paleodb.org/cgi-bin/bridge.pl?action=getTaxonomyXML&name=" + searchTerm + "&response=full");
for (int i = 0; i < paleoNameSynonyms.size(); i++) {
paleoNameSynonyms.set(i, paleoNameSynonyms.get(i) + "*");
}
}
if ((!higherTaxon.equals("*")) && (TRSynonyms != null)) {
ArrayList synonyms = new ArrayList();
String searchTerm = higherTaxon;
searchTerm = searchTerm.replace("*", "");
searchTerm = searchTerm.replaceAll(" ", "%20");
higherTaxonSynonyms = taxonomicReference.getTaxonomicSynonyms("http://paleodb.org/cgi-bin/bridge.pl?action=getTaxonomyXML&name=" + searchTerm + "&response=full");
for (int i = 0; i < higherTaxonSynonyms.size(); i++) {
higherTaxonSynonyms.set(i, higherTaxonSynonyms.get(i) + "*");
}
}
/* Get translations from XML lists */
if (!isoCountry.equals("*")) {
translationsCountry = translationsParserIsoCountry.parse("Country",isoCountry,application.getRealPath("/" + "translations/Countries.xml"));
}
if (!higherTaxon.equals("*")) {
translationsHigherTaxon = translationsParserHigherTaxon.parse("Taxon",higherTaxon,application.getRealPath("/" + "translations/Taxa.xml"));
}
/* --- Status Output ---------------------------------------------------------------------- */
/* Print the stages that have to be done */
/* The setStage JavaScript function is used to boldface the current stage; */
/* out.flush() is required to force the buffer to be flushed to the client */
/* ---------------------------------------------------------------------------------------- */
String criteria = "";
criteria += paleoName.equals("*")? "" : "ScientificName: " + paleoName + ", ";
criteria += higherTaxon.equals("*")? "" : "HigherTaxon: " + higherTaxon + ", ";
criteria += chrono.equals("*")? "" : "ChronoAttrib: " + chrono + ", ";
criteria += mineralName.equals("*")? "" : "MineralName: " + mineralName + ", ";
criteria += mineralRockGroup.equals("*")? "" : "MineralRockGroup : " + mineralRockGroup + ", ";
criteria += isoCountry.equals("*")? "" : "Country: " + isoCountry + ", ";
criteria += namedArea.equals("*")? "" : "NamedArea: " + namedArea + ", ";
criteria += locality.equals("*")? "" : "Locality: " + locality + ", ";
criteria = criteria.substring(0, criteria .lastIndexOf(","));
out.print(" " +
session.getAttribute("main.queryheader") + " " + criteria + " ");
if (translationsCountry.size() > 1) {
out.print(" Searching for Translations: " + translationsCountry + ""); }
if (translationsHigherTaxon.size() > 1) {
out.print(" Searching for Translations: " + translationsHigherTaxon + ""); }
if (paleoNameSynonyms.size() > 0) {
out.print(" Including Synonyms (Paleobiology Database): " + paleoNameSynonyms + ""); }
if (higherTaxonSynonyms.size() > 0) {
out.print(" Searching for Synonyms (Paleobiology Database): " + higherTaxonSynonyms + ""); }
out.print(" ");
%>
<%=session.getAttribute("process.message")%>
<%=session.getAttribute("process.stage1")%> |
|
<%=session.getAttribute("process.stage2")%> |
|
<%=session.getAttribute("process.stage3")%> |
|
<%=session.getAttribute("process.stage4")%> |
|
<%=session.getAttribute("process.stage5")%> |
|
<%=session.getAttribute("process.stage6")%>
<%=session.getAttribute("main.provider_preset")%>
<%out.println((String)session.getAttribute("main.krit_maxnumber") + maxResultNo + ". " +
(String)session.getAttribute("main.krit_wait1") + (waitBeforeFetch/1000) + " " + (String)session.getAttribute("main.krit_wait2"));%>
<%
out.println("" +
// (request.getHeader("User-Agent").indexOf("MSIE") == -1? "-1": "-2") + ")'>" +
" ");
%>
|